動機
直接用23的解就ok了
Problem
Merge two sorted linked lists and return it as a sorted list. The list should be made by splicing together the nodes of the first two lists.
Example 1:
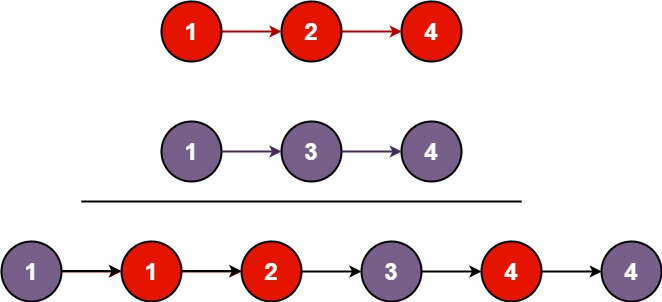
Input: l1 = [1,2,4], l2 = [1,3,4]Output: [1,1,2,3,4,4]
Example 2:
Input: l1 = [], l2 = []Output: []
Example 3:
Input: l1 = [], l2 = [0]Output: [0]
Constraints:
- The number of nodes in both lists is in the range
[0, 50]
. -100 <= Node.val <= 100
- Both
l1
andl2
are sorted in non-decreasing order.
Sol
class Solution:
def mergeKLists(self, lists: List[ListNode]) -> ListNode:
lists = [x for x in lists if x]
ret = cur = ListNode()
while lists:
tmp = min(enumerate(lists), key=lambda x: x[1].val)
cur.next = tmp[1]
cur = cur.next
if lists[tmp[0]].next is None:
del lists[tmp[0]]
else:
lists[tmp[0]] = lists[tmp[0]].next
return ret.next
def mergeTwoLists(self, l1: ListNode, l2: ListNode) -> ListNode:
return self.mergeKLists([l1,l2])