動機
真的看不懂以前寫什麼
Problem
Given the head
of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return the reordered list.
The first node is considered odd, and the second node is even, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in O(1)
extra space complexity and O(n)
time complexity.
Example 1:
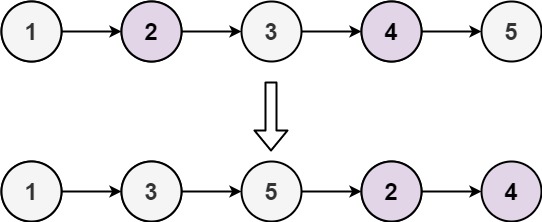
Input: head = [1,2,3,4,5]Output: [1,3,5,2,4]
Example 2:
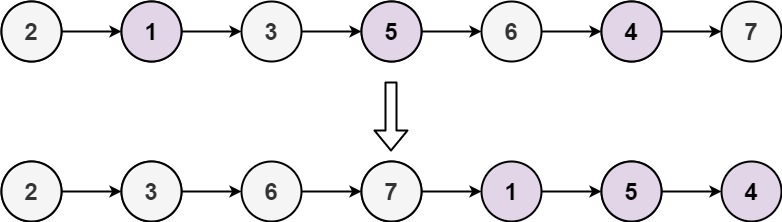
Input: head = [2,1,3,5,6,4,7]Output: [2,3,6,7,1,5,4]
Constraints:
n ==
number of nodes in the linked list0 <= n <= 104
-106 <= Node.val <= 106
Sol
善用變數去抽象
class Solution:
def oddEvenList(self, head: ListNode) -> ListNode:
if not head:
return None
# head
odd, even = head, head.next
# collect odds, evens
optr, eptr = odd, even
oprev, eprev = None, None
while optr and eptr and eptr.next: # 可以是None的前面要保證是有效的!!
onext, enext = eptr.next, eptr.next.next # 可以是None的前面要保證是有效的!!
eptr.next, optr.next = enext, onext
oprev, eprev = optr, eptr
optr, eptr = optr.next, eptr.next
# break loop
for p in [optr, eptr]:
if p:
p.next = None
# connect
if optr:
optr.next = even
else:
oprev.next = even
return odd