動機
因為題目特性,不用考慮重複走的問題
Problem
Given an m x n
integers matrix
, return the length of the longest increasing path in matrix
.
From each cell, you can either move in four directions: left, right, up, or down. You may not move diagonally or move outside the boundary (i.e., wrap-around is not allowed).
Example 1:
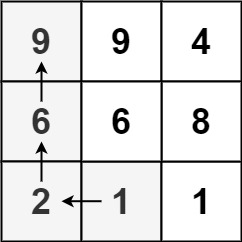
Input: matrix = [[9,9,4],[6,6,8],[2,1,1]]Output: 4Explanation: The longest increasing path is [1, 2, 6, 9]
.
Example 2:
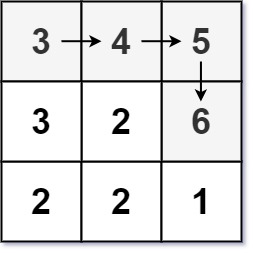
Input: matrix = [[3,4,5],[3,2,6],[2,2,1]]Output: 4Explanation: The longest increasing path is [3, 4, 5, 6]
. Moving diagonally is not allowed.
Example 3:
Input: matrix = [[1]]Output: 1
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 200
0 <= matrix[i][j] <= 231 - 1
Sol
這裡不用考慮重複走,因為數字是遞增的!! 看417
class Solution:
def longestIncreasingPath(self, ms: List[List[int]]) -> int:
@cache
def dp(i,j):
if i < 0 or i >= len(ms) or j < 0 or j >= len(ms[i]):
return 0
else:
dirs = [(i+1,j),(i-1,j),(i,j+1),(i,j-1)]
dirs = [(x,y) for (x,y) in dirs if 0 <= x < len(ms) and 0 <= y < len(ms[x]) and ms[i][j] < ms[x][y]] # 這裡不用考慮重複走,因為數字是遞增的!! 看417
dirs = [dp(x,y) for (x,y) in dirs]
if dirs:
ret = 1+max(dirs)
else:
ret = 1
return ret
return max([max([dp(i,j) for j in range(len(ms[i]))]) for i in range(len(ms))])