動機
複習dfs與交換參數的妙用
Problem
You are given two binary trees root1
and root2
.
Imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not. You need to merge the two trees into a new binary tree. The merge rule is that if two nodes overlap, then sum node values up as the new value of the merged node. Otherwise, the NOT null node will be used as the node of the new tree.
Return the merged tree.
Note: The merging process must start from the root nodes of both trees.
Example 1:
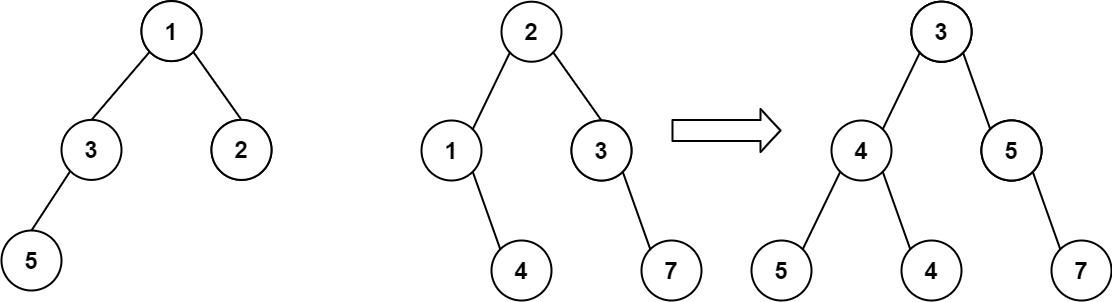
Input: root1 = [1,3,2,5], root2 = [2,1,3,null,4,null,7]Output: [3,4,5,5,4,null,7]
Example 2:
Input: root1 = [1], root2 = [1,2]Output: [2,2]
Constraints:
- The number of nodes in both trees is in the range
[0, 2000]
. -104 <= Node.val <= 104
Sol
用dfs去走,不過有趣的是用交換參數來少一些程式碼
class Solution:
def mergeTrees(self, r1: TreeNode, r2: TreeNode) -> TreeNode:
if r1 is None and r2 is None:
return None
elif r1 and r2:
ret = TreeNode(r1.val+r2.val)
ret.left = self.mergeTrees(r1.left,r2.left)
ret.right = self.mergeTrees(r1.right,r2.right)
return ret
elif r1:
ret = TreeNode(r1.val)
ret.left = self.mergeTrees(r1.left,r2)
ret.right = self.mergeTrees(r1.right,r2)
return ret
else:
return self.mergeTrees(r2,r1)