動機
dfs去點格子
Problem
Let's play the minesweeper game (Wikipedia, online game)!
You are given an m x n
char matrix board
representing the game board where:
- t
'M'
represents an unrevealed mine, t'E'
represents an unrevealed empty square, t'B'
represents a revealed blank square that has no adjacent mines (i.e., above, below, left, right, and all 4 diagonals), t- digit (
'1'
to'8'
) represents how many mines are adjacent to this revealed square, and t 'X'
represents a revealed mine.
You are also given an integer array click
where click = [clickr, clickc]
represents the next click position among all the unrevealed squares ('M'
or 'E'
).
Return the board after revealing this position according to the following rules:
- t
- If a mine
'M'
is revealed, then the game is over. You should change it to'X'
. t - If an empty square
'E'
with no adjacent mines is revealed, then change it to a revealed blank'B'
and all of its adjacent unrevealed squares should be revealed recursively. t - If an empty square
'E'
with at least one adjacent mine is revealed, then change it to a digit ('1'
to'8'
) representing the number of adjacent mines. t - Return the board when no more squares will be revealed.
Example 1:
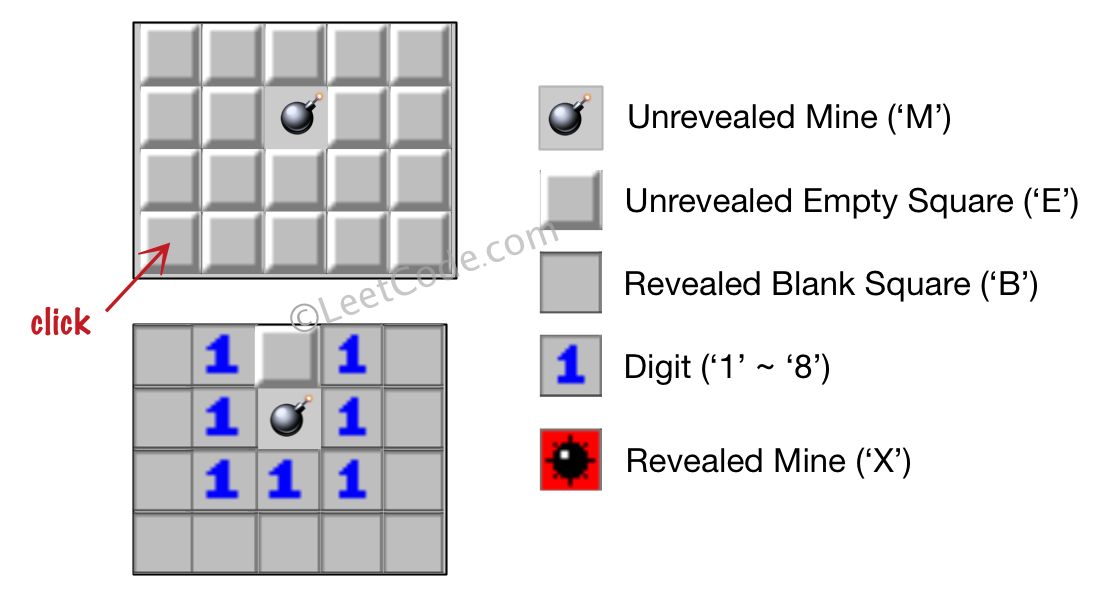
Input: board = [["E","E","E","E","E"],["E","E","M","E","E"],["E","E","E","E","E"],["E","E","E","E","E"]], click = [3,0]Output: [["B","1","E","1","B"],["B","1","M","1","B"],["B","1","1","1","B"],["B","B","B","B","B"]]
Example 2:
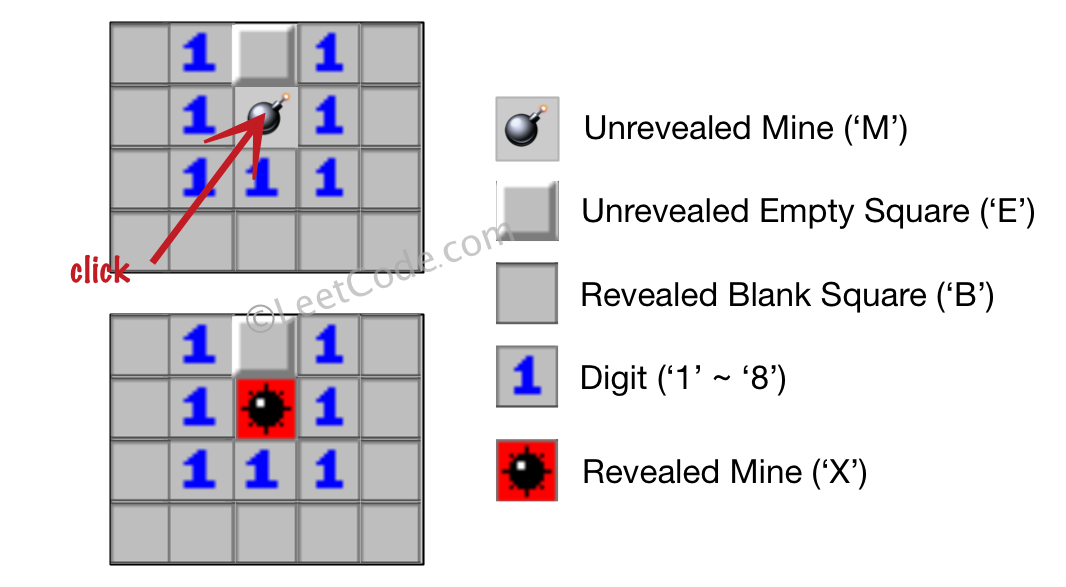
Input: board = [["B","1","E","1","B"],["B","1","M","1","B"],["B","1","1","1","B"],["B","B","B","B","B"]], click = [1,2]Output: [["B","1","E","1","B"],["B","1","X","1","B"],["B","1","1","1","B"],["B","B","B","B","B"]]
Constraints:
- t
m == board.length
tn == board[i].length
t1 <= m, n <= 50
tboard[i][j]
is either'M'
,'E'
,'B'
, or a digit from'1'
to'8'
. tclick.length == 2
t0 <= clickr < m
t0 <= clickc < n
tboard[clickr][clickc]
is either'M'
or'E'
.
Sol
在空白時用dfs去點其他格子
def surroundMine(B,i,j):
poses = [(i-1,j-1), (i-1,j), (i-1,j+1),
(i,j-1), (i,j+1),
(i+1,j-1), (i+1,j),(i+1,j+1)]
return sum([1 if B[a][b] == 'M' else 0 for (a,b) in poses if 0 <= a and a < len(B) and 0 <= b and b < len(B[a])])
def dfs(B,i,j):
if 0 <= i and i < len(B) and 0 <= j and j < len(B[i]):
if B[i][j] == 'E':
num = surroundMine(B,i,j)
if num == 0:
B[i][j] = 'B'
poses = [(i-1,j-1), (i-1,j), (i-1,j+1),
(i,j-1), (i,j+1),
(i+1,j-1), (i+1,j),(i+1,j+1)]
[dfs(B,a,b) for (a,b) in poses]
else:
B[i][j] = str(num)
class Solution:
def updateBoard(self, B: List[List[str]], c: List[int]) -> List[List[str]]:
if B[c[0]][c[1]] != 'M':
dfs(B,c[0],c[1])
else:
B[c[0]][c[1]] = 'X'
return B